Usage
Using the Android archive (*.aar) file
1- In Android Studio, create a new project or open up an existing project that you want to use the Android archive file library.
1- In the Project view, expand the libs node of the app.
3- Using the Mac Finder or Windows Explorer, select and copy the Android archive file (*.aar) e.g. mfsdk.aar.
4- Paste the aar file into the libs node in Android Studio.
5- Add the following dependencies in the app gradle file:-
implementation fileTree(dir: 'libs', include: ['*.aar'])
implementation 'com.google.code.gson:gson:2.8.5'
implementation "com.squareup.retrofit2:retrofit:2.3.0"
implementation "com.squareup.retrofit2:converter-gson:2.3.0"
6- Note:- the "master" branch developed by Kotlin language, but if you are developing by Java you should check out the second branch "javademo"
MyFaoorah Visa/Mastercard direct payment
Myfatoorah SDK now support direct payment for Visa and Master card with this feature you can implement your own design for collecting card info from the user pass them to our SDK and let us do the rest for you with only 3 easy steps your payment will be done
follow this steps to know how to use it :-
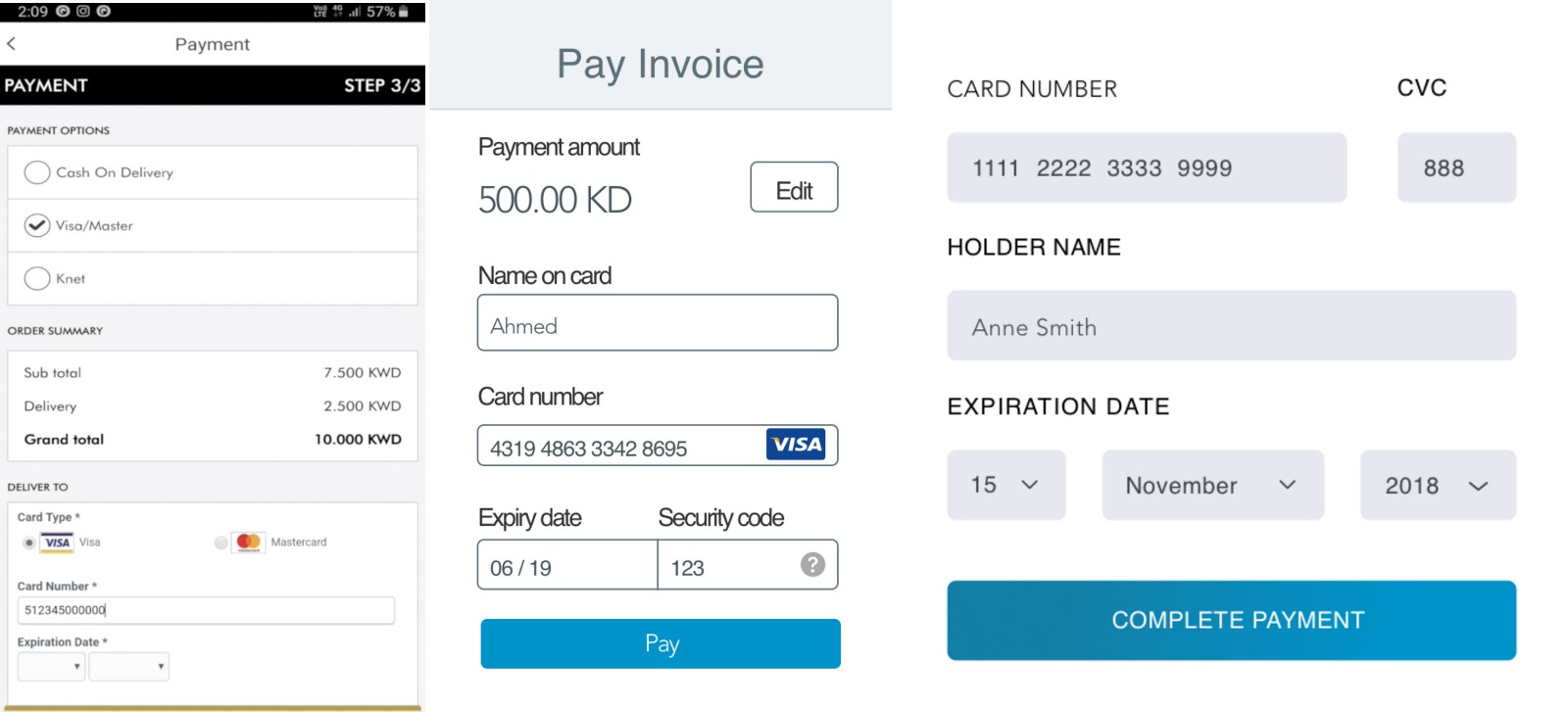
//1- Create invoice model like this:
/// - Parameters:
/// - invoiceValue: Invoice amount the customer will pay
/// - customerName: Your customer name
/// - countryCode: Your country code
/// - displayCurrency: Currency will be displayed for the user
val invoiceModel = InvoiceModel(20.0, "Test", Country.KUWAIT,
CurrencyISO.Kuwaiti_Dinar_KWD)
//2- Create Card model with card info you get from the user:
/// - Parameters:
/// - cardExpiryMonth: Card expiry month 2 digits
/// - cardExpiryYear: Card expiry year 2 digits
/// - cardSecurityCode: Card security code 3 digits
/// - cardNumber: Card number
val mfCardModel = MFCardModel("05", "21", "100", "2223000000000007")
//3- Pass them to createInvoice method and wait the response from the SDK :
/// - Parameters:
/// - invoice: Invoice model
/// - card: Card model
/// - apiLanguage: Language you prefer for getting msg from the API
MFSDK.createInvoice(this, generateInvoiceModel(), mfCardModel, "en")
InvoiceModel invoiceModel = new InvoiceModel(20.0, "Test", Country.KUWAIT, CurrencyISO.Kuwaiti_Dinar_KWD);
MFCardModel mfCardModel = new MFCardModel("05", "21", "100", "2223000000000007");
MFSDK.createInvoice(this, invoiceModel, mfCardModel, "en");
*Note : to get response from the SDK you must implement this MFSDKListener like this
class MainActivity : AppCompatActivity(), MFSDKListener{
override fun onSuccess(transactionResponseModel: TransactionResponseModel) {
val text = "Success\n\nResponse:\n\n" + Gson().toJson(transactionResponseModel)
Log.d(TAG, text)
}
override fun onFailed(statusCode: Int, error: String) {
val text = "Failed: $statusCode\n\nError Message:\n\n$error"
Log.d(TAG, text)
}
override fun onCanceled(error: String) {
Log.d(TAG, error)
}
}
public class MainActivity extends AppCompatActivity implements MFSDKListener{
@Override
public void onSuccess(@NotNull TransactionResponseModel transactionResponseModel) {
String text = "Success\n\nResponse:\n\n" + new Gson().toJson(transactionResponseModel);
Log.d(TAG, text);
}
@Override
public void onFailed(int i, @NotNull String s) {
String text = "Failed: $statusCode\n\nError Message:\n\n$error";
Log.d(TAG, text);
}
@Override
public void onCanceled(@NotNull String error) {
Log.d(TAG, error);
}
}
Updated almost 5 years ago